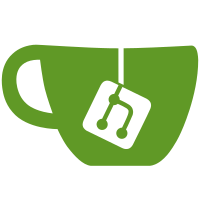
Using __init__ as the home of Watcher was causing unexpected behaviour for no good reason, so Watcher now lives in its own file as normal. There was logging configuration pertaining to processes which is not meaningful here, so removed it. Also made sure there are no unexpected log handlers.
59 lines
1.5 KiB
Python
59 lines
1.5 KiB
Python
import logging
|
|
import logging.handlers
|
|
import signal
|
|
import sys
|
|
|
|
from configparser import ConfigParser
|
|
from pathlib import Path
|
|
|
|
from .watcher import Watcher
|
|
|
|
conffile = Path('config.ini')
|
|
|
|
if not conffile.exists():
|
|
print(f"{conffile} missing, exiting")
|
|
sys.exit(1)
|
|
|
|
config = ConfigParser(empty_lines_in_values=False)
|
|
config.read(conffile)
|
|
|
|
if 'logging' not in config:
|
|
config.add_section('logging')
|
|
|
|
# Basic logging settings
|
|
baselogger = logging.getLogger('arec-watcher')
|
|
if baselogger.hasHandlers():
|
|
baselogger.handlers = []
|
|
log_level = config['logging'].get('level', 'ERROR')
|
|
baselogger.setLevel(log_level)
|
|
|
|
fmt = logging.Formatter('%(levelname)s in %(name)s: %(message)s')
|
|
|
|
stderrlog = logging.StreamHandler()
|
|
stderrlog.setLevel(log_level)
|
|
stderrlog.setFormatter(fmt)
|
|
baselogger.addHandler(stderrlog)
|
|
|
|
# Mail logging settings
|
|
if 'mail_level' in config['logging']:
|
|
from_addr = config['logging']['mail_from']
|
|
to_addr = config['logging']['mail_to']
|
|
subject = config['logging']['mail_subject']
|
|
maillog = logging.handlers.SMTPHandler('localhost',
|
|
from_addr,
|
|
[to_addr],
|
|
subject)
|
|
maillog.setLevel(config['logging']['mail_level'])
|
|
maillog.setFormatter(fmt)
|
|
baselogger.addHandler(maillog)
|
|
|
|
|
|
watch = Watcher(Path(config['arec-watcher']['watchdir']),
|
|
config['arec-watcher']['notify_url'])
|
|
watch.start()
|
|
|
|
try:
|
|
signal.pause()
|
|
except KeyboardInterrupt:
|
|
watch.shutdown()
|