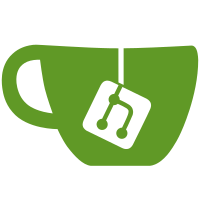
so a user will be automatically created if their email address exists in sukat but doesn't have a corresponding boka user yet.
110 lines
3.8 KiB
PHP
110 lines
3.8 KiB
PHP
<?php
|
|
class CheckoutPage extends Page {
|
|
private $userstr = '';
|
|
private $emailstr = '';
|
|
private $user = null;
|
|
|
|
public function __construct() {
|
|
parent::__construct();
|
|
if(isset($_GET['user'])) {
|
|
$this->userstr = trim(strtolower($_GET['user']));
|
|
}
|
|
if(isset($_GET['email'])) {
|
|
$this->emailstr = trim(strtolower($_GET['email']));
|
|
}
|
|
try {
|
|
$this->user = $this->user_init($this->userstr,
|
|
$this->emailstr);
|
|
} catch(Exception $e) {
|
|
$this->error = $e->getMessage();
|
|
}
|
|
}
|
|
|
|
protected function user_init($name, $email) {
|
|
$nameuser = null;
|
|
$emailuser = null;
|
|
if($name) {
|
|
try {
|
|
$nameuser = new User($name, 'name');
|
|
} catch(Exception $ue) {
|
|
# The user wasn't found locally
|
|
try {
|
|
$this->ldap->get_user($name);
|
|
$nameuser = User::create_user($name);
|
|
} catch(Exception $le) {
|
|
$err = i18n("Username {name} not found.",
|
|
$name);
|
|
throw new Exception($err);
|
|
}
|
|
}
|
|
}
|
|
if($email) {
|
|
try {
|
|
$search = $email;
|
|
if(strpos($email, '@') === false) {
|
|
$search = $email .'@dsv.su.se';
|
|
}
|
|
# Lookup email directly in ldap since we don't store it
|
|
$uid = $this->ldap->search_email($search);
|
|
} catch(Exception $le) {
|
|
$err = i18n('Email address {address} not found.',
|
|
$search);
|
|
throw new Exception($err);
|
|
}
|
|
try {
|
|
$emailuser = new User($uid, 'name');
|
|
} catch(Exception $ue) {
|
|
# User wasn't found locally, so initialize a new user
|
|
$emailuser = User::create_user($uid);
|
|
}
|
|
}
|
|
if($nameuser && $emailuser) {
|
|
if($nameuser != $emailuser) {
|
|
$err = i18n('Username and email match different users.');
|
|
throw new Exception($err);
|
|
}
|
|
return $nameuser;
|
|
}
|
|
if($nameuser) {
|
|
return $nameuser;
|
|
}
|
|
return $emailuser;
|
|
}
|
|
|
|
protected function render_body() {
|
|
$username = $this->userstr;
|
|
$email = $this->emailstr;
|
|
$displayname = '';
|
|
$notes = '';
|
|
$loan_table = '';
|
|
$subhead = '';
|
|
$enddate = '';
|
|
$disabled = 'disabled';
|
|
if($this->user !== null) {
|
|
$username = $this->user->get_name();
|
|
$email = $this->user->get_email($this->ldap);
|
|
$displayname = $this->user->get_displayname($this->ldap);
|
|
$notes = $this->user->get_notes();
|
|
$enddate = format_date(default_loan_end(time()));
|
|
$disabled = '';
|
|
$loans = $this->user->get_loans('active');
|
|
$loan_table = i18n('No active loans.');
|
|
if($loans) {
|
|
$loan_table = $this->build_user_loan_table($loans);
|
|
}
|
|
$subhead = replace(array('title' => i18n('Borrowed products')),
|
|
$this->fragments['subtitle']);
|
|
}
|
|
print(replace(array('user' => $username,
|
|
'email' => $email,
|
|
'displayname' => $displayname,
|
|
'notes' => $notes,
|
|
'end' => $enddate,
|
|
'subtitle' => $subhead,
|
|
'disabled' => $disabled,
|
|
'loan_table' => $loan_table),
|
|
$this->fragments['checkout_page']));
|
|
}
|
|
}
|
|
?>
|