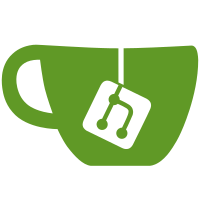
Any user who can log in via SSO but doesn't have one of the required entitlements will only ever see a listing of their own loans.
81 lines
2.8 KiB
PHP
81 lines
2.8 KiB
PHP
<?php
|
|
class PublicPage extends Page {
|
|
public function __construct() {
|
|
parent::__construct();
|
|
|
|
$user = explode('@', $_SERVER['REMOTE_USER'])[0];
|
|
try {
|
|
$this->user = new User($user, 'name');
|
|
} catch(Exception $ue) {
|
|
$this->user = User::create_user($user);
|
|
}
|
|
$this->subtitle = i18n("Hardware loans for {user}",
|
|
$this->user->get_displayname($this->ldap));
|
|
|
|
// The public page should not display a menu
|
|
$this->menuitems = array();
|
|
|
|
// This page should not require any special entitlements
|
|
$this->authorized = true;
|
|
}
|
|
|
|
protected function render_body() {
|
|
print($this->build_user_details());
|
|
}
|
|
|
|
protected function build_user_details() {
|
|
$message = '';
|
|
$hidden = 'hidden';
|
|
$active_loans = $this->user->get_loans('active');
|
|
$table_active = i18n('No active loans.');
|
|
if($active_loans) {
|
|
$table_active = $this->build_public_loan_table($active_loans);
|
|
}
|
|
$inactive_loans = $this->user->get_loans('inactive');
|
|
$table_inactive = i18n('No past loans.');
|
|
if($inactive_loans) {
|
|
$table_inactive = $this->build_public_loan_table($inactive_loans);
|
|
}
|
|
|
|
$overdue_count = count(array_filter($active_loans, function($loan) {
|
|
return $loan->is_overdue();
|
|
}));
|
|
if($overdue_count > 0) {
|
|
$message = i18n("You have {count} overdue loans.",
|
|
$overdue_count);
|
|
}
|
|
if($message) {
|
|
$hidden = '';
|
|
}
|
|
|
|
return replace(array('message' => $message,
|
|
'hidden' => $hidden,
|
|
'active_loans' => $table_active,
|
|
'inactive_loans' => $table_inactive),
|
|
$this->fragments['public_user_details']);
|
|
|
|
}
|
|
|
|
protected function build_public_loan_table($loans) {
|
|
$rows = '';
|
|
foreach($loans as $loan) {
|
|
$product = $loan->get_product();
|
|
$start = $loan->get_starttime();
|
|
$end = $loan->get_returntime();
|
|
if(!$end) {
|
|
$end = $loan->get_endtime();
|
|
}
|
|
$rows .= replace(array('status' => $loan->get_status(),
|
|
'name' => $product->get_name(),
|
|
'serial' => $product->get_serial(),
|
|
'start_date' => format_date($start),
|
|
'end_date' => format_date($end)),
|
|
$this->fragments['public_loan_table_row']);
|
|
}
|
|
return replace(array('rows' => $rows),
|
|
$this->fragments['public_loan_table']);
|
|
|
|
}
|
|
}
|
|
?>
|