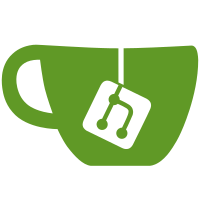
Loans are no longer displayed as a unified table, but instead each loan gets a "card" that contains the relevant data for the loan. This has had a fairly major html/css re-work as a side effect. Most views are now structured with two div-based columns so as to avoid the large blank areas that pure grid can lead to when elements are very different in height.
308 lines
4.4 KiB
CSS
308 lines
4.4 KiB
CSS
body {
|
|
font-size: 90%;
|
|
}
|
|
|
|
textarea {
|
|
height: 80px;
|
|
}
|
|
|
|
ul {
|
|
padding-left: 15px;
|
|
}
|
|
|
|
#contents {
|
|
display: grid;
|
|
grid-template-columns: [col] auto [col] auto [col];
|
|
grid-template-rows: auto [row] repeat(3, auto [row]);
|
|
grid-template-areas: "header header"
|
|
"first second"
|
|
"third fourth";
|
|
gap: 1rem;
|
|
justify-items: start;
|
|
align-items: start;
|
|
}
|
|
|
|
#message {
|
|
position: absolute;
|
|
top: 5px;
|
|
left: 50%;
|
|
transform: translate(-50%);
|
|
padding: 6px 10px;
|
|
border-style: solid;
|
|
border-width: 2px;
|
|
}
|
|
|
|
.error {
|
|
background-color: #e89e66;
|
|
border-color: #d95e00;
|
|
}
|
|
|
|
.success {
|
|
background-color: #c8cba6;
|
|
border-color: #a3a86b;
|
|
}
|
|
|
|
.tag {
|
|
margin: 1px;
|
|
background-color: #acdee6;
|
|
padding-left: 2px;
|
|
}
|
|
|
|
.label, label {
|
|
display: inline-block;
|
|
min-width: 100px;
|
|
}
|
|
|
|
.dark {
|
|
padding: 5px;
|
|
margin-bottom: 0;
|
|
background-color: #d7e0eb;
|
|
}
|
|
|
|
.light {
|
|
margin-top: 0;
|
|
padding: 5px;
|
|
background-color: #ebf0f5;
|
|
}
|
|
|
|
input[type="text"].narrow {
|
|
width: 6rem;
|
|
}
|
|
|
|
.hidden {
|
|
display: none;
|
|
}
|
|
|
|
form {
|
|
margin-bottom: 5px;
|
|
}
|
|
|
|
.column {
|
|
display: flex;
|
|
flex-direction: column;
|
|
align-items: stretch;
|
|
gap: 1rem;
|
|
}
|
|
|
|
.loan {
|
|
display: grid;
|
|
grid-template-columns: 5px 1fr;
|
|
grid-template-rows: auto auto;
|
|
grid-auto-rows: auto;
|
|
background-color: #d7e0eb;
|
|
margin-bottom: 1rem;
|
|
margin-top: 1rem;
|
|
}
|
|
|
|
.loan .status {
|
|
grid-row: 1 / -1;
|
|
grid-column: 1 / 2;
|
|
}
|
|
|
|
.loan .data {
|
|
grid-row: 1;
|
|
grid-column: 2;
|
|
}
|
|
|
|
.loan .misc {
|
|
grid-row: 2;
|
|
grid-column: 2;
|
|
justify-self: end;
|
|
padding: 2px 5px;
|
|
}
|
|
|
|
table {
|
|
border-collapse: collapse;
|
|
}
|
|
|
|
td, th {
|
|
text-align: left;
|
|
vertical-align: top;
|
|
min-width: 10px;
|
|
padding: 2px 5px;
|
|
}
|
|
|
|
td.notes {
|
|
white-space: pre-wrap;
|
|
}
|
|
|
|
.status {
|
|
width: 5px;
|
|
}
|
|
|
|
.status.available {
|
|
background-color: #a3a86b;
|
|
}
|
|
|
|
.status.on_loan, .status.active_loan {
|
|
background-color: #acdee6;
|
|
}
|
|
|
|
.status.inactive_loan {
|
|
background-color: #cdebf0;
|
|
}
|
|
|
|
.status.overdue, .status.overdue_loan {
|
|
background-color: #d95e00;
|
|
}
|
|
|
|
.status.discarded {
|
|
background-color: #a0a0a0;
|
|
}
|
|
|
|
.status.service, .status.active_service, .status.inactive_service {
|
|
background-color: #e7e08d;
|
|
}
|
|
|
|
tbody tr {
|
|
background-color: #d7e0eb;
|
|
}
|
|
|
|
tbody.single tr:nth-child(odd) {
|
|
background-color: #ebf0f5;
|
|
}
|
|
|
|
tbody.double tr:is(:nth-child(4n+1), :nth-child(4n+2)) {
|
|
background-color: #ebf0f5;
|
|
}
|
|
|
|
tbody dl {
|
|
margin: 0;
|
|
display: grid;
|
|
grid-template-columns: 2fr 5fr;
|
|
}
|
|
|
|
tbody dd {
|
|
margin: 0;
|
|
grid-column: 2;
|
|
}
|
|
|
|
thead th, tfoot tr {
|
|
background-color: #c3d1e2;
|
|
}
|
|
|
|
thead th:nth-child(2),
|
|
thead th:nth-last-child(-n+2) {
|
|
word-break: break-word;
|
|
}
|
|
|
|
input:disabled, textarea:disabled {
|
|
background-color: #ededed;
|
|
}
|
|
|
|
input[type="text"].newtag,
|
|
input[type="text"].newfield {
|
|
width: 10.5rem;
|
|
}
|
|
|
|
tbody.fixedwidth > tr > td {
|
|
min-width: 10.5rem;
|
|
}
|
|
|
|
input[type="text"].newtemplate {
|
|
width: 8rem;
|
|
}
|
|
|
|
.minibutton {
|
|
font-family: Verdana, Arial, Helvetica, sans-serif;
|
|
background-image: url(images/button-background-repeater.gif);
|
|
background-position: left;
|
|
background-repeat: repeat-x;
|
|
padding-right: 2px;
|
|
padding-left: 2px;
|
|
}
|
|
|
|
.tagremove, .termremove {
|
|
background-color: #e17e33;
|
|
padding-left: 2px;
|
|
padding-right: 2px;
|
|
color: white;
|
|
}
|
|
|
|
.tagremove:hover, .termremove:hover {
|
|
cursor: pointer;
|
|
color: white;
|
|
}
|
|
|
|
.term {
|
|
background-color: #ebf0f5;
|
|
margin: 5px;
|
|
padding-left: 2px;
|
|
}
|
|
|
|
#uploadfile {
|
|
display: none;
|
|
}
|
|
|
|
h1 {
|
|
grid-area: header;
|
|
}
|
|
|
|
#user-table {
|
|
grid-area: first;
|
|
}
|
|
|
|
#public-message {
|
|
grid-area: first / first / second / second;
|
|
}
|
|
|
|
#product-table {
|
|
grid-area: first;
|
|
}
|
|
|
|
#unseen-products {
|
|
grid-area: third;
|
|
}
|
|
|
|
#seen-products {
|
|
grid-area: fourth;
|
|
}
|
|
|
|
#inventory-register {
|
|
grid-area: second;
|
|
}
|
|
|
|
#inventory-overview {
|
|
grid-area: first;
|
|
}
|
|
|
|
#inventory-history {
|
|
grid-area: third;
|
|
}
|
|
|
|
#search {
|
|
grid-column: col 1 / col 3;
|
|
grid-row: row 1 / row 2;
|
|
justify-self: stretch;
|
|
}
|
|
|
|
#hints {
|
|
grid-column: col 1 / col 3;
|
|
grid-row: row 2 / row 3;
|
|
}
|
|
|
|
.qr {
|
|
margin: 0 10px;
|
|
padding: 5px;
|
|
border: 1px solid black;
|
|
font-weight: bold;
|
|
text-align: center;
|
|
font-size: 120%;
|
|
}
|
|
|
|
@media print {
|
|
.qr {
|
|
border: none;
|
|
}
|
|
}
|
|
|
|
.qr > a:link, .qr > a:visited {
|
|
color: black;
|
|
text-decoration: none;
|
|
}
|
|
|
|
.qr > a > * {
|
|
display: block;
|
|
margin: 0 auto;
|
|
}
|