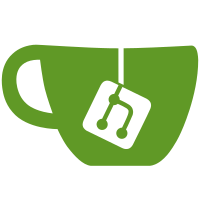
Also moved the code for creating a basic jobspec and pulling information from the relevant daisy booking into the preprocessor superclass so it can be called by both the cattura and arec preprocessors.
81 lines
3.0 KiB
Python
81 lines
3.0 KiB
Python
from abc import ABCMeta, abstractmethod
|
|
|
|
from ..queuethread import QueueThread
|
|
|
|
|
|
@QueueThread.register
|
|
class Preprocessor(QueueThread, metaclass=ABCMeta):
|
|
"""
|
|
Base class for handlers to be registered with a QueuePickup instance.
|
|
"""
|
|
def __init__(self, distributor, daisy, uldir, config):
|
|
super().__init__()
|
|
self.distributor = distributor
|
|
self.daisy = daisy
|
|
self.uploaddir_parent = uldir
|
|
self.config = config
|
|
|
|
def _process(self, job):
|
|
"""
|
|
Apply preprocessing to job and send the result to the distributor.
|
|
"""
|
|
self.distributor.put(self._preprocess(job))
|
|
|
|
def _init_jobspec(self, upload_dir=None, created=0, title=''):
|
|
jobspec = {'created': created,
|
|
'title': {'sv': title,
|
|
'en': title},
|
|
'description': '',
|
|
'presenters': [],
|
|
'courses': [],
|
|
'thumb': '',
|
|
'tags': [],
|
|
'sources': {}}
|
|
if upload_dir is not None:
|
|
jobspec['upload_dir'] = str(upload_dir)
|
|
|
|
return jobspec
|
|
|
|
def _fill_jobspec_from_daisy(self, starttime, endtime, room_id, outspec):
|
|
booking = self.daisy.get_booking(starttime, endtime, room_id)
|
|
if booking is not None:
|
|
title = {'sv': booking['displayStringLong']['swedish'],
|
|
'en': booking['displayStringLong']['english']}
|
|
outspec['title'] = title
|
|
outspec['presenters'] = booking['teachers']
|
|
if booking['description']:
|
|
outspec['description'] = booking['description']
|
|
if not outspec['presenters'] and booking['bookedBy']:
|
|
outspec['presenters'].append(booking['bookedBy'])
|
|
outspec['courses'] = booking['courseSegmentInstances']
|
|
if booking['educationalType']:
|
|
for i in booking['educationalType'].values():
|
|
# Add both english and swedish name;
|
|
# sometimes they're identical so don't add twice
|
|
if i not in outspec['tags']:
|
|
outspec['tags'].append(i)
|
|
|
|
outspec['tags'].append(
|
|
self.daisy.get_room_name(room_id))
|
|
|
|
@abstractmethod
|
|
def validate(self, queueitem):
|
|
"""
|
|
Validate a jobspec according to this preprocessor's format.
|
|
To be implemented by subclasses.
|
|
|
|
Should return True if successful, otherwise throw a KeyError or
|
|
ValueError with a descriptive message.
|
|
"""
|
|
raise NotImplementedError('validate must be implemented '
|
|
'by Preprocessor subclasses')
|
|
|
|
@abstractmethod
|
|
def _preprocess(self, job):
|
|
"""
|
|
Abstract method to implement the functionality of this preprocessor.
|
|
To be implemented by subclasses.
|
|
"""
|
|
raise NotImplementedError('_preprocess must be implemented '
|
|
'by Preprocessor subclasses')
|