Console
Basic Usages
The Proxima Console gives you a powerful command line utility to remotely inspect and control your game.
The Console comes with several built-in commands, and you can
write your own commands with unlimited potential.
Using the Console
- Type '?' to see a list of all commands.
- Type '? <command>' to see more information about a command.
- Type 'h' to see your history of commands.
- Press the Up and Down arrows to cycle through your history of commands.
- Type some characters and press Tab to cycle through auto-completion options.
Examples
// List all gameObjects starting with cube
> list cube*
Cube (1) [25654]
Cube (2) [25664]
Cube (3) [25634]
// Get the position of a specific cube
> get [25654].transform.position
Cube (1) [25654] [1, 2, 3]
// Move the cube
> move [25654] [0, 3, 0]
Moved Cube (1) [25654] to [0, 3, 0]
// Rotate the camera to look at the cube
> lookat "Main Camera" [25654].transform.position
Rotated Main Camera [23438] to [0, 3, 0]
// Add a RigidBody component to the cube
> addcomponent [25654] rigidbody
Added Rigidbody to Cube (1) [25654]
// Destroy all the cubes
> destroy cube*
Destroyed Cube (1) [25654]
Destroyed Cube (2) [25664]
Destroyed Cube (3) [25634]
// Enter fullscreen
> set screen.fullscreen true
Set Screen.fullScreen to true
// Slow down time by half
> set time.timescale 0.5
Set Time.timeScale to 0.5
// Change TextMeshPro text
> set text1.textmeshpro.text "banana phone"
Set Text1.TextMeshPro.Text to banana phone
Pattern Matching
Several built-in commands support pattern matching for gameObjects and properties:
- Use asterisk (*) to as a wildcard to when specifying gameObjects. For example: cube* will match cube1 and cube23
- Use dot notation to reference properties. For example: cube1.transform.position
- Many commands print the gameObject's ID. You can use this ID instead of the name. For example: [25654].transform.position
Types
Proxima Commands can process these types: bool, int, uint, float, string, Vector2, Vector2Int, Vector3, Vector3Int, Quaternion, Vector4, Rect, RectInt, Bounds, BoundsInt, Color, Enum, Array.
- To pass bool use true or false
- To pass string use quotes: "The quick brown fox".
- To pass Vector2, Vector3, or Vector4: [1.1, 2.2, 3.3] means new Vector3(1.1, 2.2, 3.3).
- To pass Quaternion: [10, 20, 30] means Quaternion.Euler(10, 20, 30).
- To pass Rect: [1, 2, 3, 4] means new Rect(1, 2, 3, 4).
- To pass Bounds: [1, 2, 3, 4, 5, 6] means new Bounds(new Vector3(1, 2, 3), new Vector3(4, 5, 6)).
- To pass Color use hexidecimal format: #FF0000 means red.
- You can also pass an array of the same type to a List or Array C# parameter, for example, [1, 2, 3, 4, 5].
Running Scripts
You can automate the Proxima Console to run commands in sequence. This might be helpful if you need to read many values repeatedly or setup a scene in a predefined way.
- Create a text file with a list of commands, one per line. Blank lines are ignored.
- Click "Run Script" in the console and select your file. The console will run each command in sequence.
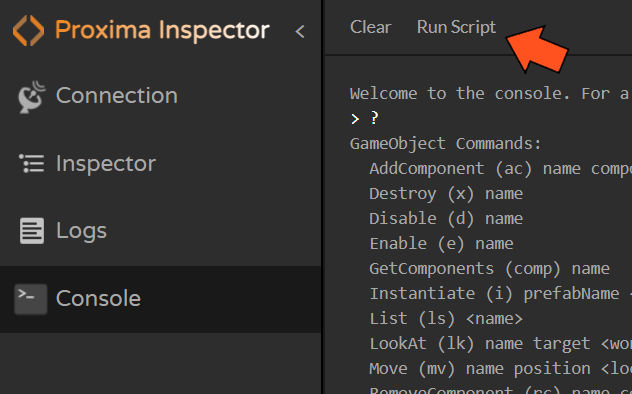